前言
之前已经写过一篇文章介绍SpringBoot整合Spring Cache,SpringBoot默认使用的是ConcurrentMapCacheManager,在实际项目中,我们需要一个高可用的、分布式的缓存解决方案,使用默认的这种缓存方式,只是在当前进程里缓存了而已。Spring Cache整合Redis来实现缓存,其实也不是一件复杂的事情,下面就开始吧。
关于Spring Cache的运用,请参考[快学SpringBoot]快速上手好用方便的Spring Cache缓存框架
新建一个SpringBoot项目
依赖
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <scope>runtime</scope> <optional>true</optional> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-configuration-processor</artifactId> <optional>true</optional> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-cache</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-redis</artifactId> </dependency> </dependencies>
|
主要是最下面两个依赖:spring-boot-starter-cache 和 spring-boot-starter-data-redis。
配置Redis
在application.properties中添加redis的配置
1 2 3
| spring.redis.host=127.0.0.1
spring.redis.port=6379
|
这是最基础的三个配置(其实默认值就是这样,就算不写也可以)。当然,还有空闲连接数,超时时间,最大连接数等参数,我这里都没有设置,在生产项目中,根据实际情况设置。
RedisCacheConfig
新建RedisCacheConfig.class
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| @Configuration @EnableCaching public class RedisCacheConfig {
@Bean public CacheManager cacheManager(RedisConnectionFactory redisConnectionFactory) { RedisCacheWriter redisCacheWriter = RedisCacheWriter.nonLockingRedisCacheWriter(redisConnectionFactory); RedisSerializer<Object> jsonSerializer = new GenericJackson2JsonRedisSerializer(); RedisSerializationContext.SerializationPair<Object> pair = RedisSerializationContext.SerializationPair .fromSerializer(jsonSerializer); RedisCacheConfiguration defaultCacheConfig=RedisCacheConfiguration.defaultCacheConfig() .serializeValuesWith(pair); return new RedisCacheManager(redisCacheWriter, defaultCacheConfig); }
}
|
如果要设置缓存管理器所管理的缓存名字,RedisCacheManager构造方法提供一个可变参数的构造器:
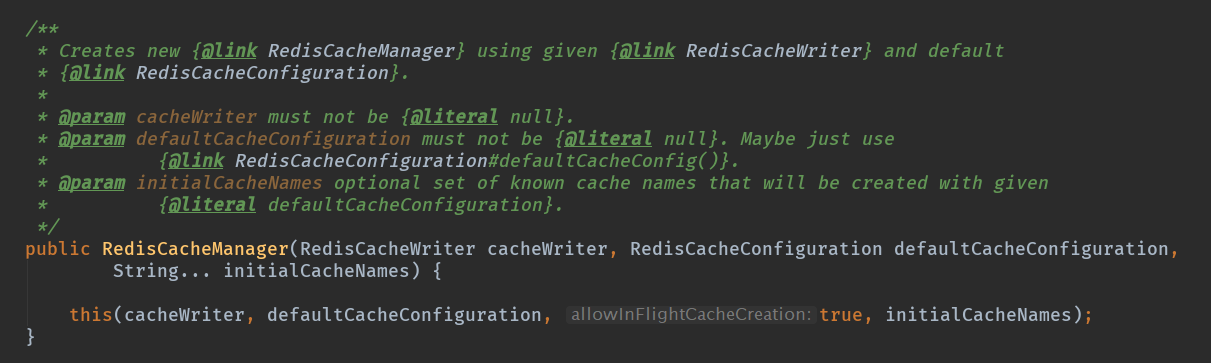
测试
新建一个MockService.java,代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| @Service public class MockService {
@Cacheable(value = "listUsers", key = "#username") public List<String> listUsers(String username) { System.out.println("执行了listUsers方法"); return Arrays.asList("Happyjava", "Hello-SpringBoot", System.currentTimeMillis() + ""); }
}
|
新建一个TestController.java,代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| @RestController public class TestController {
private final MockService mockService;
public TestController(MockService mockService) { this.mockService = mockService; }
@GetMapping(value = "/listUsers") public Object listUsers(String username) { return mockService.listUsers(username); }
}
|
请求接口:
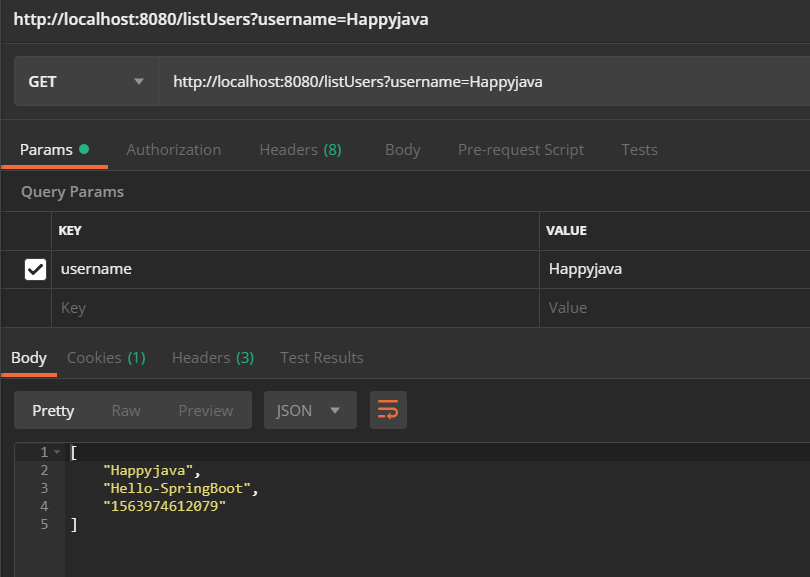
Redis中的数据:
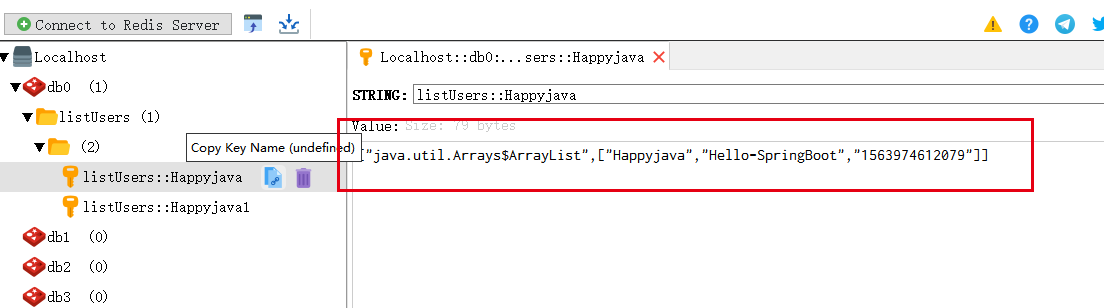